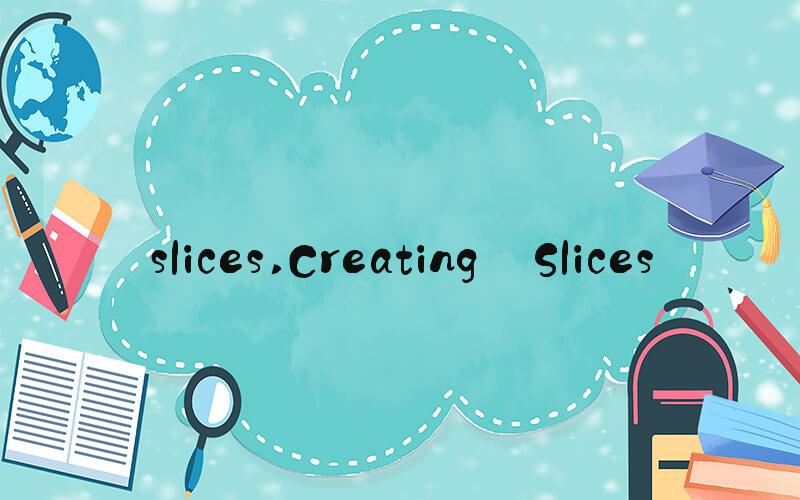
Slices are an essential feature of Go programming language. They are dynamically-sized arrays that store a sequence of elements of a particular type. Slices can be used to manage large datasets, sort and manipulate data, and implement algorithms.
Creating SlicesTo create a slice, we use the make() function. The syntax is as follows:
s := make([]type, length, capacity)
Here, type
refers to the data type of the elements in the slice, length
is the number of elements in the slice, and capacity
is the maximum number of elements the underlying array can hold.
We can add new elements to a slice using the append()
function. The syntax is simple:
s = append(s, element)
This statement appends the element
to the end of s
.
To copy a slice, we use the copy()
function. The syntax is as follows:
copy(destination, source)
Here, destination
is the slice where we want to copy the elements, and source
is the slice from where we want to copy.
Slices can be used to perform various operations on data. Here are some of the common operations:
Sorting: We can sort a slice using the sort
package.
Filtering: We can filter a slice using the filter
function.
Reversing: We can reverse a slice using the reverse
function.
Searching: We can search for an element in a slice using the indexOf
function.
Slices are a powerful feature in Go. They provide a flexible and efficient way to manage and manipulate data. Whether you are working with large datasets or small arrays, slices can help you achieve your goals with ease.