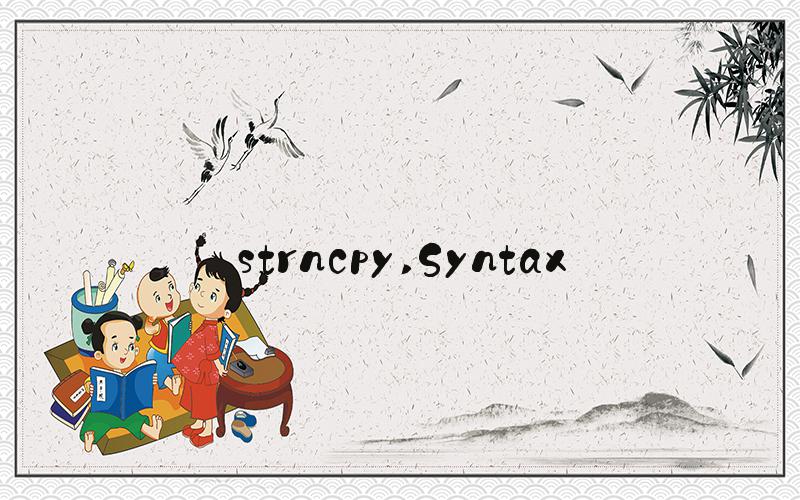
Strncpy is a function in C programming language that is used to copy a string from one location to another specified location for a given amount of characters. The string is copied until either the specified number of characters have been copied or the original string has been completely copied. This function can be useful in many different types of programs, especially those that involve manipulating strings in some way.
SyntaxThe syntax for strncpy is:
char *strncpy(char *destination, const char *source, size_t num);
The parameters for the strncpy function include:
destination: This is the pointer to the destination array where the content is to be copied. This array should be large enough to hold the copied content.
source: This is the pointer to the source of data to be copied.
num: This is the maximum number of characters to be copied from the source string.
Example 1The following example shows how strncpy can be used to copy a string to a new location:
#include <stdio.h>
#include <string.h>
int main() {
char string1[20] = "Hello";
char string2[20];
strncpy(string2, string1, 3);
printf("%s", string2);
return 0;
}
In this example, the string "Hello" is copied to string2, but only the first 3 characters are copied. Therefore, string2 will contain "Hel".
Example 2Another example that demonstrates the usefulness of strncpy is to ensure that the destination string is null-terminated. If the size of the destination array is smaller than the length of the source string, then the copied string will not be null-terminated. To ensure that the destination string is null-terminated, the following code can be used:
#include <stdio.h>
#include <string.h>
int main() {
char string1[20] = "Hello";
char string2[4];
strncpy(string2, string1, 3);
string2[3] = '\0';
printf("%s", string2);
return 0;
}
In this example, the size of string2 is only 4 bytes, meaning that only the first 3 characters of string1 will be copied. To prevent any issues due to lack of null-termination, a null character is added manually by using the statement string2[3] = '\0';
ConclusionThe strncpy function is a useful tool for copying and manipulating strings in C programming language. It is important to use this function properly to avoid issues such as not null-terminating the destination string or copying too many characters. By understanding the syntax and using examples like the ones provided, programmers can utilize strncpy effectively in their programs.